1. Introduction
C language is widely used in system programming and embedded system development. Among its fundamental components, operators play a crucial role in performing basic operations.
In this article, we will provide a comprehensive explanation of C language operators, covering everything from basic usage to advanced applications, error handling, and optimization techniques.
By mastering operators, you can write efficient programs, minimize errors, and enhance the overall performance of your code.
In the later sections, we will also discuss error handling techniques and optimization strategies for operators, offering practical insights that are useful in real-world development.
By the end of this article, you will gain confidence in using C language operators effectively.
2. Basics and Types of Operators
What Are Operators?
Operators are symbols used to manipulate data in C language.
C provides various types of operators, including arithmetic operators, assignment operators, comparison operators, and logical operators.
These operators are essential for constructing program logic.
Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations.
+
(Addition):a + b
→ Adds two numbers.-
(Subtraction):a - b
→ Subtracts the second number from the first.*
(Multiplication):a * b
→ Multiplies two numbers./
(Division):a / b
→ Divides the first number by the second.%
(Modulo):a % b
→ Returns the remainder ofa
divided byb
.
Assignment Operators
Assignment operators are used to assign values to variables.
=
(Assignment):a = 5
→ Assigns 5 to variablea
.+=
(Addition Assignment):a += 2
→ Adds 2 toa
and assigns the result back toa
.-=
(Subtraction Assignment):a -= 1
→ Subtracts 1 froma
and assigns the result back toa
.
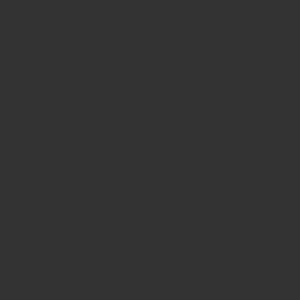
Comparison Operators
Comparison operators compare two values and return either true or false.
==
(Equal to):a == b
→ Returns true ifa
is equal tob
.!=
(Not equal to):a != b
→ Returns true ifa
is not equal tob
.>
(Greater than):a > b
→ Returns true ifa
is greater thanb
.
Logical Operators
Logical operators are used to evaluate multiple conditions.
&&
(Logical AND):a && b
→ Returns true if botha
andb
are true.||
(Logical OR):a || b
→ Returns true if eithera
orb
is true.
sizeof
Operator
The sizeof
operator is used to determine the memory size of a variable or data type.
It is particularly useful for optimizing memory usage in structures and arrays.
int size = sizeof(int); // Typically, int is 4 bytes
It is also helpful in determining the size of structures and arrays.
struct example {
int a;
char b;
};
int size = sizeof(struct example); // Retrieves the size of the structure
You can also use sizeof
to calculate the number of elements in an array.
int arr[10];
int num_elements = sizeof(arr) / sizeof(arr[0]); // Calculates the number of elements in the array
3. Detailed Explanation and Examples of Each Operator
Examples of Arithmetic Operators
#include <stdio.h>
int main() {
int a = 10;
int b = 3;
printf("Addition: %dn", a + b);
printf("Subtraction: %dn", a - b);
printf("Multiplication: %dn", a * b);
printf("Division: %dn", a / b);
printf("Modulo: %dn", a % b);
return 0;
}
This code demonstrates the basic use of arithmetic operators.
Note that when performing division with integers, the decimal part is truncated.
4. Operator Precedence and Associativity
Operator precedence determines the order in which operations are performed when multiple operators are used in a single expression.
Associativity (left-to-right or right-to-left) defines the order of evaluation for operators with the same precedence.
Example of Precedence
int a = 2 + 3 * 4; // Result is 14
In this expression, multiplication has higher precedence than addition, so the result is 14
.
If you want addition to be performed first, use parentheses to change the order.
int a = (2 + 3) * 4; // Result is 20
Operator Precedence Table
Precedence | Operators |
---|---|
High | ++ , -- (Increment, Decrement) |
Medium | * , / , % (Multiplication, Division, Modulo) |
Low | + , - (Addition, Subtraction) |
Explanation of Associativity
In C, the assignment operator =
is right-associative, which means the rightmost assignment is evaluated first.
int a, b, c;
a = b = c = 5; // Assigns 5 to all variables
Since the assignment operator is right-associative, c = 5
is executed first, followed by b = c
, and finally a = b
.
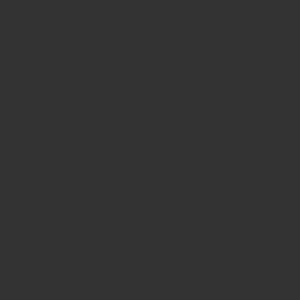
5. Advanced Operators: Bitwise and Shift Operators
Bitwise Operators
Bitwise operators are used to manipulate individual bits of a number.
They are commonly used in low-level programming and embedded systems.
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int result = a & b; // Result is 0001 (1)
In this example, the bitwise AND operator (&
) calculates the logical AND of corresponding bits from both numbers.
Shift Operators
Shift operators move bits to the left or right, effectively performing multiplication or division by powers of two.
int a = 5; // 0101 in binary
int result = a << 1; // 1010 (10) - equivalent to multiplying a by 2
The left shift operator (<<
) is commonly used for efficient multiplication in performance-critical applications.
6. Error Handling and Optimization for Operators
Handling Division by Zero
Dividing by zero is mathematically undefined and causes a program crash in C.
It is essential to check for zero before performing division.
#include <stdio.h>
int main() {
int a = 10;
int b = 0;
if (b != 0) {
printf("Result: %dn", a / b);
} else {
printf("Error: Division by zero is not allowed.n");
}
return 0;
}
In this example, the program checks if b
is zero before executing the division to prevent a crash.
Handling Overflow
Integer overflow occurs when the result of an operation exceeds the maximum value a variable type can hold.
Since C does not automatically detect overflow, you must check for it manually.
#include <stdio.h>
#include <limits.h> // To use INT_MAX
int main() {
int a = INT_MAX;
int b = 1;
if (a > INT_MAX - b) {
printf("Error: Overflow detected.n");
} else {
int result = a + b;
printf("Result: %dn", result);
}
return 0;
}
This code checks if adding b
to a
would exceed INT_MAX
before performing the operation.
Optimizing Operations
Operator optimization is particularly useful in performance-sensitive environments.
Using bitwise shift operators instead of multiplication or division can improve execution speed.
int a = 5;
int result = a << 1; // Equivalent to a * 2
Bitwise operations are computationally faster than arithmetic operations, making them beneficial in embedded systems and low-level programming.
7. Conclusion
In this article, we covered a wide range of topics related to C language operators, from basic usage to advanced techniques like error handling and optimization.
Understanding operator precedence, associativity, and potential errors such as division by zero or overflow is crucial for writing robust code.
Key takeaways:
– **Operators** form the foundation of program logic in C.
– **Operator precedence and associativity** determine the order of execution in complex expressions.
– **Error handling** prevents common issues like division by zero and overflow.
– **Optimization techniques** such as bitwise operations can enhance performance in critical applications.
### **Next Steps**
To further enhance your C programming skills, consider learning about pointers, arrays, and memory management.
Mastering these topics will enable you to write more efficient and scalable code.