1. What is extern
?
In C language, extern
is a keyword that signals you’re “borrowing” a variable or function that was defined in another file. For instance, if you want to use a global variable defined in one file from another file, you would use extern
. When a program is split across multiple files, extern
helps share data between them.
Think of it like this: your program is a house with many rooms, and extern
is like a “borrowing agreement” that lets you use a tool from the room next door. It means, “This item isn’t here, but you can find it in another file.”
Example
// file1.c
int g_data = 100;
// file2.c
extern int g_data;
void printData() {
printf("%dn", g_data); // Accesses g_data defined in file1.c
}
In this example, g_data
is defined in file1.c
, and by using extern
, file2.c
is able to access that variable.
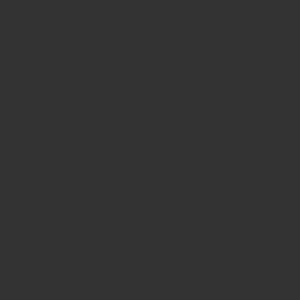
2. Relationship Between extern
and Global Variables
extern
is closely related to global variables. A global variable, once defined, can be accessed from any file in the program. However, other files don’t automatically know about its existence. That’s where extern
comes in — it tells other files, “Hey, this variable exists somewhere else!”
One important thing to note: extern
is only a declaration, not a definition. It does not allocate memory. The actual memory for the variable is allocated in the original file where it is defined.
Example of a Global Variable
// file1.c
int counter = 0;
// file2.c
extern int counter; // Refers to counter defined in another file
void incrementCounter() {
counter++; // Updates the value of counter
}
3. Using extern
in Header Files
In large-scale projects, repeatedly declaring variables or functions in every file can be tedious. That’s where header files come in handy. A header file is a centralized place to store information that needs to be shared across multiple files. By placing extern
declarations in a header file, you make it easy for other files to refer to the same global variables or functions.
You can think of a header file as a “toolbox” for the entire project. It gathers shared tools in one place, so that other files can simply include it and use what they need.
Example of a Header File
// globals.h
extern int global_variable;
void printGlobalVariable();
// file1.c
#include "globals.h"
int global_variable = 10;
// file2.c
#include "globals.h"
void printGlobalVariable() {
printf("%dn", global_variable);
}
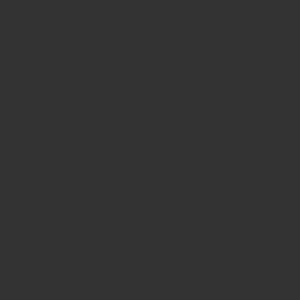
4. Common Mistakes with extern
There are a few important points to keep in mind when using extern
. For example, you cannot initialize a variable when using extern
. That’s because extern
is only a declaration — not a definition. Also, if you declare a variable with extern
in a file where the actual definition doesn’t exist, the program will result in a linker error.
Typical Mistake
extern int data = 100; // Initialization is not allowed here
As shown above, trying to initialize a variable with extern
will cause an error. extern
is used to reference a variable defined in another file, not to define or initialize it.
5. Practical Use Cases of extern
extern
is especially useful in large-scale projects. For example, in programs that span multiple modules, you can declare shared data or functions using extern
, and reuse them across different files.
This approach promotes modularity in your program, making the codebase easier to maintain and understand. Each file can function independently while still sharing necessary data through extern
declarations.
Practical Example
// main.c
#include "globals.h"
int main() {
printGlobalVariable(); // Calls a function defined in another file
return 0;
}
// globals.c
#include "globals.h"
int global_variable = 100;
void printGlobalVariable() {
printf("%dn", global_variable); // Prints the global variable
}
This article has covered everything from the basics of extern
to practical usage. Understanding how extern
works is essential for splitting and reusing code in C programming.