Introduction
If you’re working with the C programming language, you simply can’t ignore the static
keyword. This small keyword has a huge impact on variable lifecycles and function scope. Like a behind-the-scenes director, it quietly but powerfully controls important aspects of your code. In this article, we’ll dive into how to use static
effectively and share practical best practices. And don’t worry—we’ll keep things light with a bit of humor along the way!
1. What is static
in C?
The static
keyword in C is used with variables and functions to extend a variable’s lifecycle and limit the scope of variables and functions. Normally, a variable is destroyed when a function ends, but if you declare it as static
, it retains its value until the program terminates. In a way, static
is like a stubborn character who says, “Once I’m set, I’m sticking around!”
Using static
, you can create “limited scope” variables and functions that are accessible only within the same file. This helps prevent naming conflicts in modular programs.
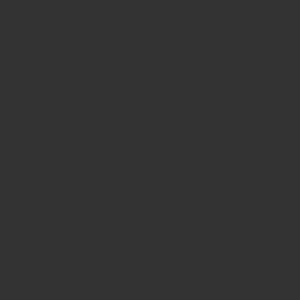
2. Static Variables: Local and Global
2.1 Local Static Variables
When you declare a local variable as static
, it is initialized only once and retains its value between function calls. This makes it perfect for situations where you want to preserve state within a function—like a counter, for example.
void count() {
static int counter = 0;
counter++;
printf("Counter: %d\n", counter);
}
int main() {
count(); // Output: Counter: 1
count(); // Output: Counter: 2
return 0;
}
2.2 Global Static Variables
A global static
variable can only be accessed within the file in which it is declared. This helps prevent accidental access from other files and avoids naming conflicts in large projects. It’s a useful way to manage variables cleanly within each module.
// file1.c
static int globalVar = 100;
void printGlobalVar() {
printf("GlobalVar: %d\n", globalVar);
}
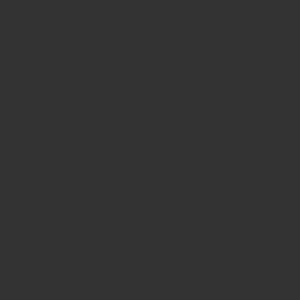
3. Static Functions: Limiting Scope
When you declare a function as static
, its scope is limited to the file where it’s defined. This makes it ideal for helper functions that you don’t want to expose outside the file. It allows you to control which functions are publicly accessible, leading to safer and more modular code design.
// file1.c
static void helperFunction() {
printf("This is a helper function\n");
}
void publicFunction() {
helperFunction();
printf("This is a public function\n");
}
4. Cautions When Using static
The biggest thing to watch out for when using static
is how variables are initialized. A static
variable is initialized only once at the start of the program and is never re-initialized. So if you mistakenly try to reinitialize it every time a function is called, you’ll lose the main benefit of using static
.
void resetStaticVar() {
static int num = 5;
num = 10; // Resetting it every time defeats the purpose of using static
}
5. Best Practices for Using static
To make the most of the static
keyword, keep the following best practices in mind:
- Use
static
with local variables to maintain state between function calls. - Always declare helper functions as
static
if they don’t need to be accessed from other files. - Use
static
with global variables to limit their scope and prevent name conflicts between modules.
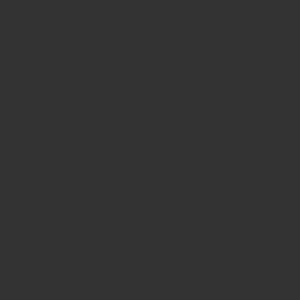
6. Performance Impact of static
Variables
static
variables remain in memory for the entire duration of the program. While this allows for faster access since the memory allocation only happens once, it also means they continuously occupy memory. If overused, this can increase memory consumption, so it’s important to use them wisely. On the plus side, avoiding frequent memory allocation and deallocation can improve performance in some cases.
Conclusion
In C programming, the static
keyword is a powerful tool for managing variable lifecycles and function scope. When used correctly, it helps you write cleaner, more maintainable, and robust code. However, if misused, it can lead to unexpected bugs or wasted memory. Be sure to use static
with a clear understanding and follow best practices to get the most benefit from it.