- 1 1. What is volatile in C Language?
- 2 2. Understanding the Purpose of volatile
- 3 3. How volatile Works in Embedded Systems
- 4 4. Using volatile in Multithreaded Environments
- 5 5. Common Misunderstandings About volatile
- 6 6. Best Practices for Using volatile
- 7 7. Using volatile Effectively for Reliable Code
1. What is volatile
in C Language?
The volatile
keyword in C tells the compiler, “Hey, treat this variable a bit differently!” Normally, the compiler performs optimizations to make your program run more efficiently. However, volatile
prevents certain optimizations. Why would you want to do that? Because some variables can change due to external factors.
For example, variables that receive data from hardware sensors or variables that can be changed by other threads in a multithreaded environment. If the compiler optimizes these variables, it could lead to unexpected bugs or behavior. By declaring them as volatile
, you’re telling the compiler: “Always read this variable’s latest value!”
By the way, it’s a bit amusing that the literal translation of volatile
in Japanese means “evaporative”—like the variable disappears into thin air! But in reality, it’s just a technique to make sure you always get the up-to-date value.
2. Understanding the Purpose of volatile
The purpose of volatile
is to ensure that the program does not overlook changes to a variable that may be modified outside the program itself—such as by hardware or an external system. For instance, sensor values or hardware registers might get updated repeatedly inside a program loop.
Normally, the compiler may optimize variables that appear unchanged in a loop by caching their values. But when you use volatile
, you’re instructing the compiler to fetch the value directly from memory every time it’s accessed.
volatile int sensor_value;
while (1) {
// Ensures the sensor value is read correctly each time
printf("Sensor value: %dn", sensor_value);
}
In this example, without volatile
, the compiler might cache the sensor value, causing it to print the same value repeatedly. By adding volatile
, you guarantee that the program reads the latest sensor value each time it runs through the loop.
3. How volatile
Works in Embedded Systems
volatile
plays a particularly important role in embedded systems. In such systems, it’s common to directly monitor hardware states or interact with sensors and actuators. Therefore, it’s critical to handle variables that can change in real time correctly.
For example, variables used for hardware registers or within interrupt service routines (ISRs) are often modified outside the main program flow. If you don’t use volatile
, the compiler may cache these variables, causing the program to miss real-time updates from the hardware.
volatile int interrupt_flag;
void interrupt_handler() {
interrupt_flag = 1; // Set the flag when an interrupt occurs
}
int main() {
while (!interrupt_flag) {
// Wait for the interrupt flag to be set
}
printf("Interrupt occurred!n");
return 0;
}
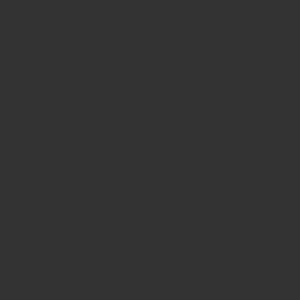
4. Using volatile
in Multithreaded Environments
In multithreaded programs, there are situations where volatile
can be helpful. However, it’s important to understand that volatile
does not guarantee synchronization between threads. All it does is prevent the compiler from caching the variable—it does not make operations thread-safe (e.g., atomic).
volatile
is commonly used for shared flag variables between threads. But for more complex synchronization, you need to use other mechanisms like mutexes or semaphores.
volatile int shared_flag = 0;
void thread1() {
// Modify the flag in Thread 1
shared_flag = 1;
}
void thread2() {
// Detect flag change in Thread 2
while (!shared_flag) {
// Wait for the flag to be set
}
printf("Flag detected!n");
}
5. Common Misunderstandings About volatile
There are many common misconceptions about the use of volatile
. One of the most frequent is the belief that using volatile
can ensure synchronization between threads. In reality, volatile
does not handle synchronization or mutual exclusion between threads.
It’s also important to understand that volatile
does not disable all optimizations. For example, incrementing or decrementing a volatile
variable is not atomic. In a multithreaded environment, operations on volatile
variables can lead to race conditions and unpredictable behavior.
volatile int counter = 0;
void increment_counter() {
counter++; // This operation is NOT atomic!
}
6. Best Practices for Using volatile
Here are some best practices for using volatile
correctly and effectively:
- Always use it for hardware access: When dealing with hardware registers or external inputs, use
volatile
to ensure that your program always reads the most up-to-date value. - Don’t use it for thread synchronization: Since
volatile
is not a thread synchronization mechanism, use mutexes or semaphores when working with complex multithreaded operations. - Avoid unnecessary use: Overusing
volatile
can lead to performance issues or unexpected behavior. Only use it when it’s truly needed.
7. Using volatile
Effectively for Reliable Code
volatile
plays a critical role in programming for hardware and multithreaded environments, but it must be used with a clear understanding. When applied appropriately, it helps improve the reliability of your code. However, it’s equally important to know its limitations and avoid relying on it for things it’s not designed to handle.