- 1 1. Introduction
- 2 2. What Is a Switch Statement in C?
- 3 3. Basic Syntax of the Switch Statement
- 4 4. Example Use Case: Menu Selection with Switch
- 5 5. Switch vs. If Statements: Which One Should You Use?
- 6 6. Common Mistakes and How to Avoid Them
- 7 7. Advanced Use: Switch Statements with Enums
- 8 8. Summary
1. Introduction
The switch statement is a powerful and convenient tool commonly used in programming to simplify conditional branching. In the C programming language, using a switch statement helps organize multiple options more clearly, making the code easier to read and reducing the chances of bugs. In this article, we’ll walk through the basics and practical uses of the switch statement in C, including actual code examples.
2. What Is a Switch Statement in C?
A switch statement is a control structure that compares a specific variable against multiple values and executes the corresponding block of code. It is especially useful when conditions are defined by numbers or enumerated types (enum
).
For example, you can use a switch statement to write a clean and simple program that performs different actions based on a user’s menu selection.
switch (condition) {
case value1:
// Process for value1
break;
case value2:
// Process for value2
break;
default:
// Process if no value matches
}
In this syntax, the expression inside switch
is evaluated, and the code block matching the appropriate case
is executed. If none of the cases match, the default
block runs, allowing you to handle unexpected values gracefully.
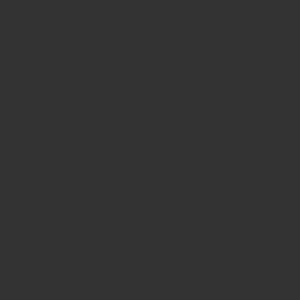
3. Basic Syntax of the Switch Statement
Let’s look at a basic example. The following program displays a message based on the value of a dice roll.
int main(void) {
int dice = 3;
switch (dice) {
case 1:
printf("Rolled a 1.");
break;
case 2:
printf("Rolled a 2.");
break;
case 3:
printf("Rolled a 3.");
break;
case 4:
printf("Rolled a 4.");
break;
case 5:
printf("Rolled a 5.");
break;
case 6:
printf("Rolled a 6.");
break;
default:
printf("Invalid roll.");
break;
}
return 0;
}
In this example, if the value of dice
is 3, the program prints “Rolled a 3.” If an invalid value is given, the default
block will handle it by displaying “Invalid roll,” ensuring proper error handling.
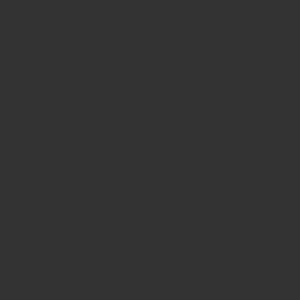
4. Example Use Case: Menu Selection with Switch
The switch statement is especially effective when handling different actions based on user input. In the following example, the program displays a message corresponding to the selected food item.
int main(void) {
int choice;
printf("Menu:");
printf("1. Hamburger");
printf("2. Pizza");
printf("3. Pasta");
printf("Please select a number: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("You selected Hamburger.");
break;
case 2:
printf("You selected Pizza.");
break;
case 3:
printf("You selected Pasta.");
break;
default:
printf("Invalid selection.");
break;
}
return 0;
}
This program shows a different message depending on the user’s menu choice. By using a switch
statement, the code becomes simpler, more readable, and easier to maintain.
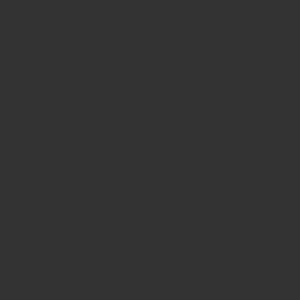
5. Switch vs. If Statements: Which One Should You Use?
Both switch
and if
statements are used for conditional branching, but there are key differences in when each is more appropriate.
When to use a switch statement:
- When you’re comparing fixed values and branching based on them (e.g., menu selection, status codes).
- When the conditions are simple and you want to perform different actions based on specific values.
When to use an if statement:
- When the condition involves more complex logic (e.g., range checks, inequalities).
- When conditions are based on logical expressions or involve multiple variables.
While switch
is great for straightforward value-based conditions, if
offers more flexibility for handling complex expressions. It’s important to choose the right one depending on the situation.
6. Common Mistakes and How to Avoid Them
Here are some common mistakes when using switch
statements and how to prevent them.
1. Forgetting the break
statement (causing fall-through)
If you omit the break
statement, the code will continue executing into the next case even if it doesn’t match. This is called a “fall-through.” Unless you intend this behavior, always include a break
at the end of each case
block.
2. Not using the default
case
When there’s a possibility of user input or unexpected values, including a default
case helps handle errors more safely. This reduces the risk of the program behaving unpredictably.
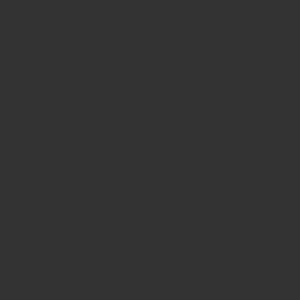
7. Advanced Use: Switch Statements with Enums
One powerful way to enhance your use of switch
statements is by combining them with enums (enumerated types). Enums let you define meaningful names instead of raw numbers, making your code more intuitive and easier to understand.
enum Fruit { APPLE, BANANA, ORANGE };
int main(void) {
enum Fruit fruit = BANANA;
switch (fruit) {
case APPLE:
printf("You selected Apple.");
break;
case BANANA:
printf("You selected Banana.");
break;
case ORANGE:
printf("You selected Orange.");
break;
default:
printf("Invalid selection.");
break;
}
return 0;
}
In this example, an enum
is used to define the fruit options, and a switch
statement branches based on the selected fruit. Using enums in this way improves both the readability and maintainability of your code.
8. Summary
In C programming, the switch
statement is highly effective when dealing with conditions based on specific values. Compared to if
statements, switch
is better suited for simple value-based branching. It allows you to write efficient code while maintaining readability.
Make use of switch
statements in your programming to create cleaner, more structured code—especially in situations where multiple distinct options need to be handled. With thoughtful use, your code will become more elegant and easier to maintain.