1. Introduction
In programming, looping structures are essential for executing tasks efficiently. In the C language, there are several types of loops, and among them, the while loop is ideal when the number of repetitions is not known in advance. This article explains the basics and practical use cases of the while loop in C, in a way that’s easy to understand even for beginners. Through real code examples, you’ll learn the advantages of while loops and how to apply them in your own projects.
2. What Is a while Loop in C?
A while loop is a control structure that repeatedly executes a block of code as long as a specified condition remains true. When the condition becomes false, the loop stops. The following example shows the basic syntax of a while loop.
int i = 0;
while (i < 10) {
printf("The value of i is: %d\n", i);
i++; // Increment the counter
}
In this example, the value of i
is printed repeatedly as long as it’s less than 10. Once i
reaches 10, the loop ends. The while loop is particularly useful when the number of iterations is not known beforehand, as it allows dynamic repetition based on conditions.
3. When to Use a while Loop
A while loop is especially useful when the number of repetitions isn’t known in advance. It’s perfect for situations like waiting for the user to type “exit” or continuously checking input from an external sensor. The following example shows a program that keeps accepting numbers from the user until a negative number is entered.
int value = 0;
while (value >= 0) {
printf("Please enter a number: ");
scanf("%d", &value);
printf("You entered: %d\n", value);
}
This program continues to run as long as the user enters a non-negative number. Once a negative value is entered, the loop ends. In scenarios where the number of repetitions can’t be predicted, a while loop provides a highly flexible solution.
4. Common Mistakes with while Loops
One of the most common mistakes when using a while loop is creating an infinite loop. This happens when the loop’s exit condition is never met. Here’s a classic example:
int i = 0;
while (i < 10) {
printf("The value of i is: %d\n", i);
// Forgot to increment i!
}
In this code, the value of i
never changes, so the condition i < 10
remains true forever. As a result, the loop runs infinitely and never exits. Infinite loops can put a heavy load on your system, so it’s important to always ensure that the exit condition will eventually be satisfied.
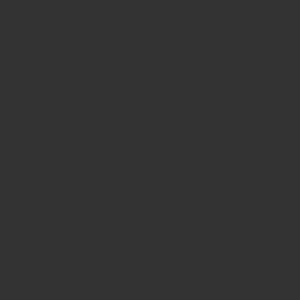
5. Practical Examples of while Loops
Let’s take a look at a few real-world examples that use while loops.
Example 1: Repeating User Input
This program continues to prompt the user for a message until they type “終了” (which means “exit” in Japanese).
char input[50];
while (strcmp(input, "終了") != 0) {
printf("Enter a message (type 終了 to quit): ");
scanf("%s", input);
printf("You entered: %s\n", input);
}
Example 2: Repeated Addition
This program keeps adding numbers until the total exceeds 100.
int sum = 0;
int num = 1;
while (sum < 100) {
sum += num;
num++;
printf("Current total: %d\n", sum);
}
These examples demonstrate how while loops can be used to perform flexible and repetitive tasks based on dynamic conditions.
6. Advanced Usage of while Loops
The while loop can also be applied to more complex tasks. The following example uses nested while loops to process a two-dimensional array.
int i = 0, j = 0;
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
while (i < 3) {
j = 0;
while (j < 3) {
printf("%d ", matrix[i][j]);
j++;
}
printf("\n");
i++;
}
This code uses nested while loops to display the contents of a 3×3 matrix. As you can see, while loops are powerful tools for handling more complex data structures and processes.
7. Comparing while Loops with Other Loop Structures
C provides several types of loop structures besides the while loop. The table below compares the most common types and when to use each one.
Loop Type | Best Use Case | Key Features |
---|---|---|
while loop | When the number of repetitions is unknown | Repeats while the condition is true; the condition is checked before each iteration |
for loop | When the number of repetitions is known | Initializes, checks the condition, and updates the counter all in one line |
do-while loop | When the code needs to run at least once | Condition is checked after the loop body; guarantees at least one execution |
This comparison can help you choose the most appropriate loop structure depending on the specific requirements of your program.
8. Conclusion
In this article, we explored the while loop in the C programming language in detail. While loops allow you to perform repeated actions based on dynamic and flexible conditions, making them suitable for a wide range of programming scenarios. Just be careful to avoid infinite loops by ensuring that your exit conditions are properly set. Try out the code examples in this article to experience the power and versatility of while loops for yourself, and strengthen your programming skills in the process!