1. What is const
in C Language?
When writing programs in C, the const
keyword is useful for preventing variables from being accidentally modified. It’s a way to tell the compiler, “This value should not change!” Once a value is assigned to a const
variable, it cannot be modified later.
For example, take a look at the following code:
const int x = 10;
x = 20; // Error!
By using const
like this, the value of x
is locked in place, preventing accidental changes. It’s like stopping a variable from “breaking free,” helping you keep your program more stable and reliable.
2. Why Should You Use const
?
2.1 Improve Code Safety
Using const
helps reduce the risk of accidentally modifying important variables or data. For instance, if you unintentionally change a critical configuration value or constant, it could destabilize your entire program. const
prevents this by making sure such values stay unchanged.
2.2 Enable Compiler Optimizations
When the compiler knows a value won’t change—thanks to const
—it can optimize your code more effectively. const
variables are easier to cache, which can lead to performance improvements, especially when they’re used frequently.
2.3 Improve Readability and Team Collaboration
Using const
is a best practice that enhances code readability. In team development environments, it clearly communicates that “this value will not change,” reducing the chances of other developers unintentionally modifying the variable. It adds an extra layer of clarity and protection to your codebase.
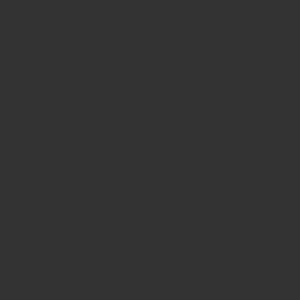
3. Understanding the Relationship Between Pointers and const
The relationship between pointers and const
can be confusing, especially for beginners learning C. The behavior changes depending on where the const
keyword is placed.
const int *p;
In this case, the value pointed to byp
(that is,*p
) cannot be changed, but the pointerp
itself can point to a different address.int *const p;
Here, the pointerp
cannot be changed—it must always point to the same address. However, the value at that address (*p
) can be modified.const int *const p;
In this case, neither the pointerp
nor the value it points to can be changed. It’s a truly “read-only” situation.
Pointers are powerful tools, but they can easily lead to bugs if used incorrectly. Pay close attention to where you place const
when working with pointers.
4. Practical Use Cases for const
4.1 Using const
in Function Parameters
When passing arrays or pointers to a function, use const
if the function should not modify the data. For example, the following code ensures that the array contents remain unchanged inside the function:
void printArray(const int arr[], int size) {
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("
");
}
This allows the function to safely access the array without the risk of accidental modification.
4.2 Using const
for Lookup Tables
For data that doesn’t need to change during program execution—such as lookup tables—it’s common to use const
. In the following example, the names of the days are stored as fixed strings:
const char *days[] = {"Monday", "Tuesday", "Wednesday"};
This ensures the data remains constant and reliable throughout the program.
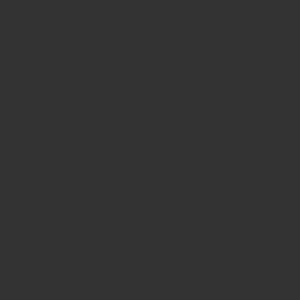
5. Misuses and Pitfalls of const
5.1 Common Mistakes When Using const
If you misuse const
, it can lead to unexpected behavior or compilation errors. Take a look at the following example:
void modifyArray(const int arr[], int size) {
arr[0] = 10; // Compilation error!
}
In this function, we try to modify an element of the array arr
, which is marked as const
. This causes a compilation error because const
guarantees that the data cannot be changed. Forcing a change will break that promise, and the compiler will complain.
5.2 Be Careful with Casting Away const
While it’s technically possible to remove the const
qualifier using const_cast
, doing so is risky. It may lead to undefined behavior, especially when working with system-level code or when the data is truly meant to be read-only. As a general rule, you should avoid casting away const
and respect its purpose for writing safe and reliable code.
6. Conclusion
In C programming, the const
keyword is a powerful tool for improving code safety and optimizing performance. By properly using const
with variables and pointers, you can write more stable code and prevent bugs before they happen.
Next time you write a program, try using const
proactively. You might be surprised how often you realize, “Oh, I could’ve used const
here too!” It’s a small change that can make a big difference in the quality and maintainability of your code.