- 1 1. Introduction
- 2 2. What is malloc?
- 3 3. Basic Usage of malloc
- 4 4. The Importance of Releasing Memory with free()
- 5 5. The Importance of Checking for NULL
- 6 6. The Difference Between malloc and calloc
- 7 7. Practical Example: Dynamically Allocating Memory for Strings with malloc
- 8 8. Using malloc with Structures
- 9 9. Common Mistakes When Using malloc
- 10 10. Summary
1. Introduction
When you start programming in C, you’ll often begin by using arrays to manage memory. However, as your programs become more complex, you’ll likely encounter situations where you need more flexible memory management. This is where “dynamic memory allocation” becomes essential. The malloc
function is a key tool for this—it allows you to allocate memory dynamically during the execution of a program.
To put it simply, malloc
is like ordering food made on demand, while fixed-size arrays are more like a buffet. With malloc
, you “order” only the amount of memory you need, and when you’re done, you “clear the plate” by freeing the memory using the free
function. In this article, we’ll take a closer look at how malloc
works and how to use it effectively in your C programs.
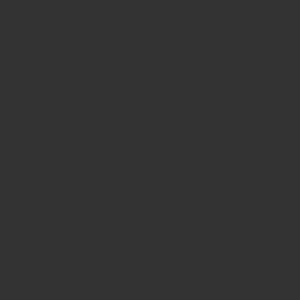
2. What is malloc
?
malloc
stands for “memory allocation” and is a function in C used to allocate memory dynamically. During program execution, it allocates a specified amount of memory and returns a pointer to the beginning of that block. This allows you to use only as much memory as needed while your program runs, enabling more flexible memory management compared to fixed-size arrays.
In actual code, malloc
is used like this:
int *array = (int*)malloc(10 * sizeof(int));
In this example, memory is allocated for an array of 10 integers. One important point is that malloc
returns a pointer to the beginning of the allocated memory as a void*
type, which may not match the expected data type. Therefore, it’s common to cast it to the appropriate type. In this case, (int*)
is used to cast it to an integer pointer.
3. Basic Usage of malloc
Now, let’s take a closer look at how to use malloc
in practice. The basic syntax of malloc
is as follows:
void* malloc(size_t size);
The malloc
function takes a single argument: the number of bytes of memory you want to allocate. It then reserves that amount of memory and, if successful, returns a pointer to the beginning of the allocated block. The return type is void*
, which is a generic pointer type that can be cast to any other pointer type. For example, you can use it like this:
int *array = (int*)malloc(10 * sizeof(int));
Here, sizeof(int)
is used to calculate how much memory is needed for 10 integers. This approach ensures that the correct amount of memory is allocated, even across different environments or systems. It’s important to remember that once you’re done using the allocated memory, you must free it using the free
function. Otherwise, you could run into a problem known as a memory leak.
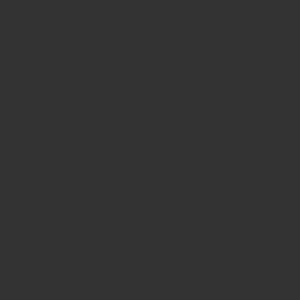
4. The Importance of Releasing Memory with free()
Dynamic memory allocation is incredibly useful, but there’s one important thing to keep in mind: you must always free any memory you’ve allocated. If you forget to do this, it can lead to memory leaks, where your program consumes memory unnecessarily and never gives it back to the system.
Memory allocated with malloc
can be released using the free()
function, like this:
free(array);
If memory is not freed, it will remain reserved as a system resource until the program ends. In long-running programs, this can become a serious issue. Think of it like borrowing plates from a kitchen—if you don’t return them (i.e., call free
), the kitchen will eventually run out of clean plates.
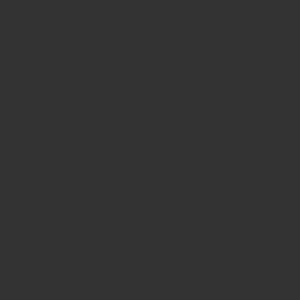
5. The Importance of Checking for NULL
The malloc
function returns NULL
if it fails to allocate memory. This can happen, for example, if you try to allocate more memory than the system can provide. When using malloc
, it’s essential to check whether the returned pointer is NULL
to ensure that the memory was successfully allocated. This is a crucial part of writing safe and reliable code.
int *array = (int*)malloc(100000000 * sizeof(int));
if (array == NULL) {
// Handle memory allocation failure
printf("Memory allocation failed.\n");
return 1;
}
By performing this kind of check, you can handle memory allocation errors gracefully. Adding just a small safeguard like this can help prevent major problems later on in your application.
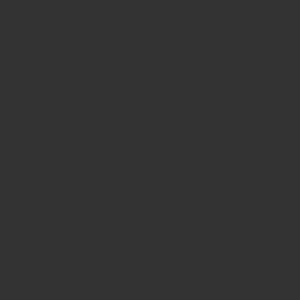
6. The Difference Between malloc
and calloc
In addition to malloc
, C provides other functions for dynamic memory allocation. One of them is calloc
. While malloc
and calloc
are very similar, there are a few important differences. malloc
allocates a block of memory of the specified size, but does not initialize its contents. On the other hand, calloc
not only allocates memory but also initializes all bits to zero.
How to Use calloc
int *array = (int*)calloc(10, sizeof(int));
This code allocates memory for an array of 10 integers and initializes each element to zero. The main difference compared to malloc
is that calloc
takes two arguments: the number of elements and the size of each element. This syntax makes it easier to allocate memory for arrays or data structures with multiple elements.
Which one you should use depends on the situation. If you need the memory to be initialized to zero, calloc
is the better choice. If you don’t need initialization and want better performance, malloc
may be more appropriate.
7. Practical Example: Dynamically Allocating Memory for Strings with malloc
Let’s look at a practical example of using malloc
to dynamically allocate memory for strings. In C, strings are typically handled using fixed-size character arrays. However, when the length of a string is only known at runtime, or when you want to manipulate strings dynamically, malloc
becomes very useful.
char *str = (char*)malloc(50 * sizeof(char));
if (str == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
sprintf(str, "Hello, World!");
printf("%s\n", str);
free(str);
In this example, memory is allocated for 50 characters, and the string “Hello, World!” is stored in that space. Don’t forget to free the memory using the free
function after you’re done using it. With malloc
, you can manage memory for strings in a much more flexible way than with fixed-size arrays.
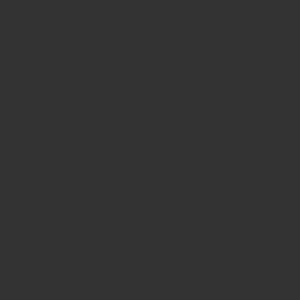
8. Using malloc
with Structures
Next, let’s look at how to use malloc
to dynamically allocate memory for a structure. Structures are powerful data types that allow you to group different kinds of data together. You can also allocate memory for them dynamically, just like arrays or strings.
typedef struct {
int id;
char *name;
} Person;
Person *p = (Person*)malloc(sizeof(Person));
if (p == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
p->name = (char*)malloc(50 * sizeof(char));
sprintf(p->name, "John Doe");
p->id = 1;
printf("ID: %d, Name: %s\n", p->id, p->name);
free(p->name);
free(p);
In this example, we dynamically allocate memory for a Person
structure and then separately allocate memory for its name
member. This demonstrates how each member of a structure can also be dynamically allocated using malloc
, allowing for more flexible and scalable memory management.
9. Common Mistakes When Using malloc
Let’s go over some common mistakes that beginners often make when using malloc
. By avoiding these pitfalls, you can write safer and more efficient programs.
- Forgetting to free allocated memory
If you forget to free memory allocated withmalloc
usingfree()
, it will result in a memory leak. This can be especially problematic in programs that run for a long time. No matter how complex your program is, always make it a habit to release memory once you’re done using it. - Omitting
NULL
checks
It’s easy to forget thatmalloc
can fail and returnNULL
. Always check forNULL
immediately after attempting to allocate memory, and include proper error-handling code. - Accessing uninitialized memory
Memory allocated withmalloc
is not initialized. If you try to use it without initializing it first, your program may behave unpredictably. If you need initialized memory, consider usingcalloc
instead.
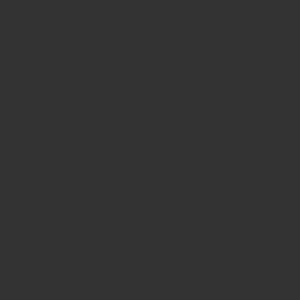
10. Summary
malloc
is a powerful tool in C for allocating memory dynamically. However, to use it effectively, you need a solid understanding and proper memory management practices. From the basic usage to advanced applications like working with structures and strings, mastering malloc
will help you write more flexible and efficient C programs. The next time you write code, remember to “order” memory with malloc
and “return the plate” by freeing it once you’re done!
FAQ
- What should I do if
malloc
fails to allocate memory?
Ifmalloc
fails, it returnsNULL
. Always check forNULL
and implement appropriate error-handling routines to ensure your program remains stable. - Which should I use:
malloc
orcalloc
?
If you don’t need the memory to be initialized, usemalloc
. If you want the allocated memory to be zero-initialized,calloc
is the better choice.