1. What is memset
? Overview and Use Cases
memset
is a memory manipulation function used in the C programming language. It is used to initialize a block of memory with a specific value. This function sets each byte in the specified memory block to a given value, making it an efficient way to clear or initialize memory. It is commonly used for initializing arrays or clearing sensitive data for security purposes.
- Example: Initializing arrays, clearing sensitive data, etc.
Using this function properly can improve memory management efficiency and enhance the security of your program.
2. Basic Usage of the memset
Function
2.1 Syntax of memset
The basic syntax of memset
is as follows:
#include <string.h>
void *memset(void *buf, int ch, size_t n);
- First argument (
buf
): Specifies the starting address of the memory block to initialize. - Second argument (
ch
): The value to be set in memory. It is stored byte by byte. - Third argument (
n
): The number of bytes to be set in memory.
2.2 Example Usage of memset
The following is a basic example of initializing a portion of an array with a specific value:
#include <stdio.h>
#include <string.h>
int main() {
char buf[10] = "ABCDEFGHIJ";
// Write '1' to 3 bytes starting from the 3rd byte
memset(buf + 2, '1', 3);
printf("buf string → %s\n", buf); // Output: "AB111FGHIJ"
return 0;
}
In this example, memset
is used to fill 3 bytes starting from the 3rd byte of the buffer buf
with the character '1'
. The resulting output is "AB111FGHIJ"
, showing the targeted section has been replaced with '1'
.
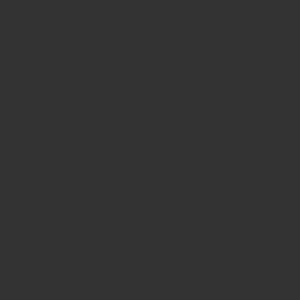
3. Practical Use Cases of memset
3.1 Initializing Arrays
memset
is convenient when initializing arrays. You can simplify the initialization process by filling the entire array with a specific value. The following is an example of initializing an array with zeros:
#include <stdio.h>
#include <string.h>
int main() {
int arr[10];
memset(arr, 0, sizeof(arr));
return 0;
}
In this example, the entire arr
array is initialized to zero.
3.2 Clearing Memory for Security
memset
is also used to clear sensitive data from memory, such as passwords or encryption keys. The following example demonstrates how to use memset
to clear a password:
#include <string.h>
void clearPassword(char *password) {
// Process involving the password
memset(password, 0, strlen(password)); // Clear password with zeros
}
By ensuring that the password does not remain in memory, this approach helps improve security.
3.3 Combining with Dynamic Memory Allocation
You can also use memset
to initialize memory that was dynamically allocated with malloc
. Here’s an example:
#include <stdlib.h>
#include <string.h>
int main() {
char *buffer = (char *)malloc(50);
if (buffer == NULL) {
return 1; // Memory allocation failed
}
// Initialize memory with zeros
memset(buffer, 0, 50);
free(buffer); // Free allocated memory
return 0;
}
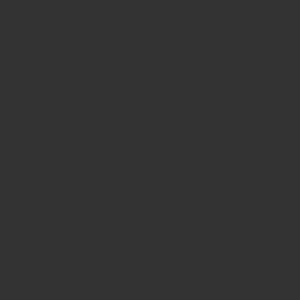
4. Precautions When Using memset
4.1 Preventing Buffer Overflows
When using memset
, it’s important to avoid buffer overflows. If the specified size exceeds the allocated memory block, it may overwrite other memory regions. Always use the sizeof
operator to ensure the correct size is specified.
char buffer[10];
memset(buffer, 0, sizeof(buffer)); // Correct size specification
4.2 Effects on Data Types
Because memset
operates on a byte-by-byte basis, using it to initialize arrays of integers or floating-point values with anything other than zero can lead to unexpected results. This is especially true when structures contain members of different data types. Use caution in these cases.
4.3 Handling Compiler Optimization
When using memset
to clear sensitive data, such as passwords, there’s a risk that the compiler might optimize away the memset
call. To prevent this, consider using the volatile
keyword or a secure alternative like memset_s
.
volatile char *secure_clear = memset(password, 0, strlen(password));
5. Comparison Between memset
and Other Memory Functions
5.1 Difference from memcpy
While both memset
and memcpy
are memory manipulation functions, they serve different purposes.
memset
: Initializes a memory block with a specific value. It sets a single value byte by byte.memcpy
: Copies data from one memory block to another. It is used for copying arbitrary data, not for initialization.
5.2 Comparison with a for
Loop
Both memset
and a for
loop can be used to initialize arrays, but each has its pros and cons.
- Advantages of
memset
: The code is concise and easy to read. It is usually faster than afor
loop due to compiler optimizations. - Advantages of
for
loop: Offers flexible initialization, such as assigning different values to each element.
int array[5];
for (int i = 0; i < 5; i++) {
array[i] = i; // Set different values to each element
}
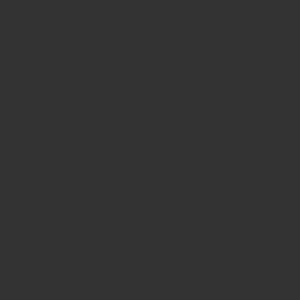
6. Summary
memset
is a powerful tool for efficiently initializing and clearing memory. However, it’s important to understand how to use it properly—especially when it comes to specifying the correct size and being aware of its effects on different data types. When used correctly, memset
can help improve both the performance and security of your programs.