- 1 1. Introduction | What is printf?
- 2 2. Basic Syntax of printf
- 3 3. Understanding Format Specifiers
- 4 4. Controlling Output | Field Width and Precision
- 5 5. Advanced Features | Flags and Format Options
- 6 6. Common Mistakes and How to Avoid Them
- 7 7. Practical Example | Combining Everything
- 8 8. Summary
- 9 9. We’d Love Your Feedback
1. Introduction | What is printf
?
When you start learning the C programming language, one of the first functions you’ll encounter is printf
. This function is used to output text and variable values to the console. It’s an essential tool for checking how your program behaves and is widely used for debugging.
#include <stdio.h>
int main(void) {
printf("Hello, World!\n");
return 0;
}
Hello, World!
is an iconic piece of code that marks your first step into the world of programming. It’s often the very first program beginners write — and it’s a perfect way to start learning the basics of printf
.
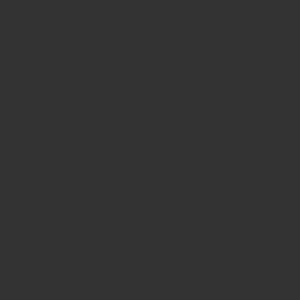
2. Basic Syntax of printf
printf
is a powerful function that lets you easily display text and data. Here’s a basic example of how it works:
printf("Hello, world!\n");
In the example above, the message "Hello, world!"
will be displayed in the console, followed by a line break due to the \n
(newline) character. In C, output does not automatically move to a new line, so it’s important to add newline characters manually when needed.
3. Understanding Format Specifiers
printf
supports a variety of data types by using format specifiers. These special codes tell the function how to format and display different types of data. Here are some of the most commonly used specifiers:
%d
: Displays an integer.%f
: Displays a floating-point number (up to 6 decimal places by default).%s
: Displays a string.%c
: Displays a single character.
Example: Outputting Multiple Data Types
int age = 25;
float height = 175.5;
char initial = 'A';
char name[] = "Taro";
printf("Name: %s\nAge: %d\nHeight: %.1f\nInitial: %c\n", name, age, height, initial);
The output will look like this:
Name: Taro
Age: 25
Height: 175.5
Initial: A
As you can see, you can combine multiple format specifiers to display different types of data in a single output.
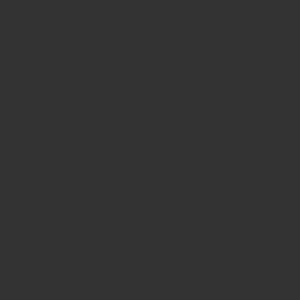
4. Controlling Output | Field Width and Precision
With printf
, you can fine-tune the output format by specifying field width and precision.
Field Width
Field width sets the minimum number of characters to be printed. The following code prints a number with a total width of 5 characters:
printf("%5d", 123);
The output will be:
123
Precision
Precision is used to control the number of digits after the decimal point for floating-point numbers:
printf("%.2f", 3.14159);
This will produce the following output:
3.14
5. Advanced Features | Flags and Format Options
printf
also supports flags that give you more control over how the output is formatted.
Left Alignment and Zero Padding
To left-align the output, use the -
flag. To pad numbers with leading zeros, use the 0
flag:
printf("%-5d", 123); // Left-aligned
printf("%05d", 123); // Zero-padded
Output:
123
00123
Hexadecimal and Octal Output
You can also use printf
to display numbers in hexadecimal or octal formats:
printf("%x", 255); // Hexadecimal
printf("%o", 255); // Octal
The output will be:
ff
377
These formats are especially useful in system programming and debugging.
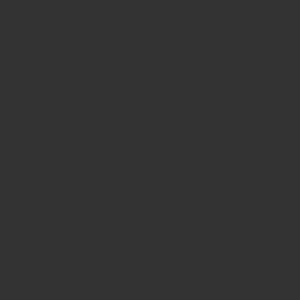
6. Common Mistakes and How to Avoid Them
One of the most common mistakes when using printf
is mismatching format specifiers with data types. For example, using a floating-point format specifier for an integer will result in an error or unexpected behavior:
int age = 25;
printf("%f", age); // Incorrect: age is an integer
To avoid issues, make sure that the format specifier matches the type of data you’re printing. In this case, use %d
for integers.
7. Practical Example | Combining Everything
Now let’s look at a full example that puts everything we’ve learned into practice:
#include <stdio.h>
int main() {
printf("Name: %-10s Age: %3d\n", "Alice", 30);
printf("Price: %7.2f\n", 123.456);
return 0;
}
This program will output the following:
Name: Alice Age: 30
Price: 123.46
As you can see, by using format specifiers, field width, precision, and flags together, you can create neatly formatted and easy-to-read output.
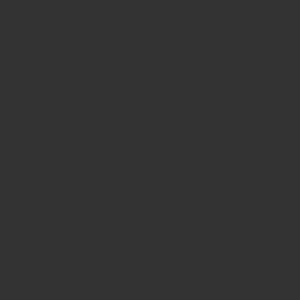
8. Summary
printf
is a powerful and flexible function in C that allows you to customize output formatting with ease. By mastering format specifiers, field width, precision, and flags, you can create clean, structured output. These skills are especially useful when debugging or checking your program’s behavior. Make sure to apply what you’ve learned from this guide in your own coding projects!
9. We’d Love Your Feedback
If you have any questions or need further clarification after reading this guide, feel free to leave a comment below. Your feedback helps us improve and provide even better content in the future. Thank you for reading!